Hi all,
I need to know how can I make a delay of ~1msec in that platform.
beside the Order sleep() that gives me delay in seconds.
Thanks,
How can I make a delay in Visual Studio 2010
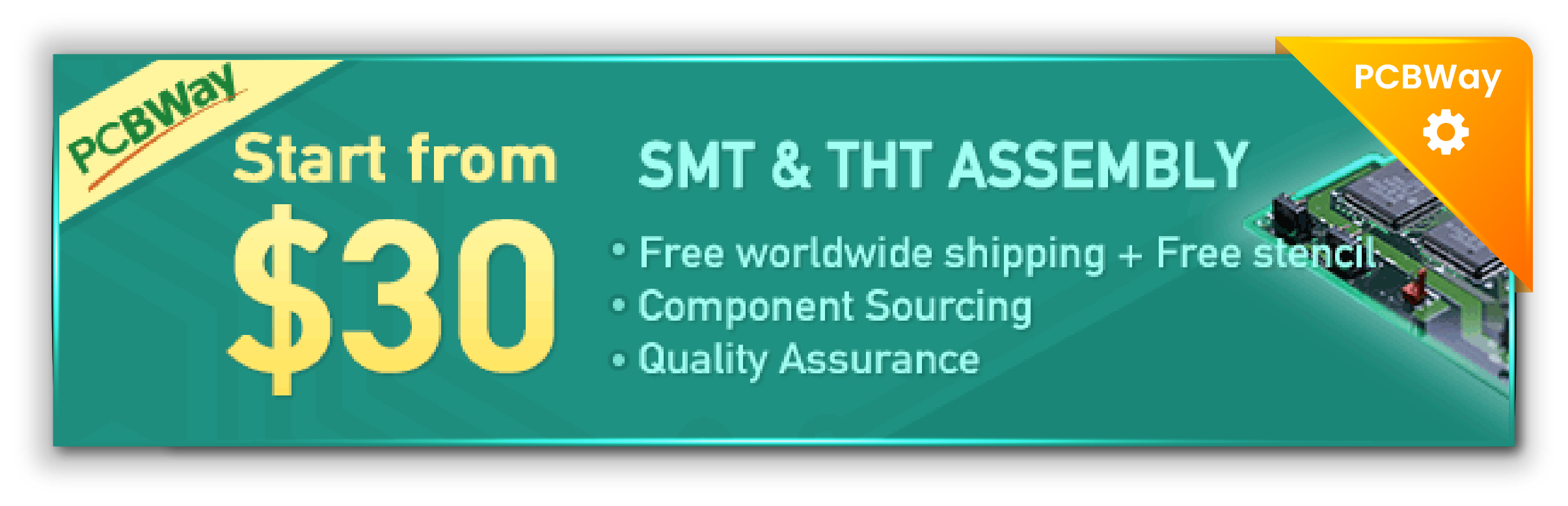
[code]// header files here #include <windows.h> int _tmain(int argc, _TCHAR* argv[]) { // code Sleep(1); return 0; }If you are using Windows Application then follow this:
1. Define a timer ID on top of your cpp file
[code]#define MY_TIMER_ID (WM_USER + 200)2. Declare a timer variable inside your class definition in header file
[code]class MainDlg : public CDialogEx { // defs UINT_PTR m_MyTimer; }3. Implement your timer function,
[/code]
4. Start your timer for example in OnInitDialog()[code]void MainDlg::OnTimer(UINT_PTR nIDEvent) { // use nIDEvent to detect if you have multiple timers // your code CDialogEx::OnTimer(nIDEvent); }[/code]
5. Kill your timer when you are done. Example follows,[code]BOOL MainDlg::OnInitDialog() { CDialogEx::OnInitDialog(); // Code m_MyTimer = SetTimer(MY_TIMER_ID, 1, NULL); }[/code]
Read More:[code]void MainDlg::OnClose( ) { // Code if (!KillTimer (m_MyTimer)) MessageBox(_T("Could not kill timer!")); CDialogEx::OnClose(); }[url=http://www.theengineeringprojects.com/2013/10/how-to-add-delay-in-microsoft-visual.html]HOW TO ADD A DELAY IN VISUAL STUDIO 2010