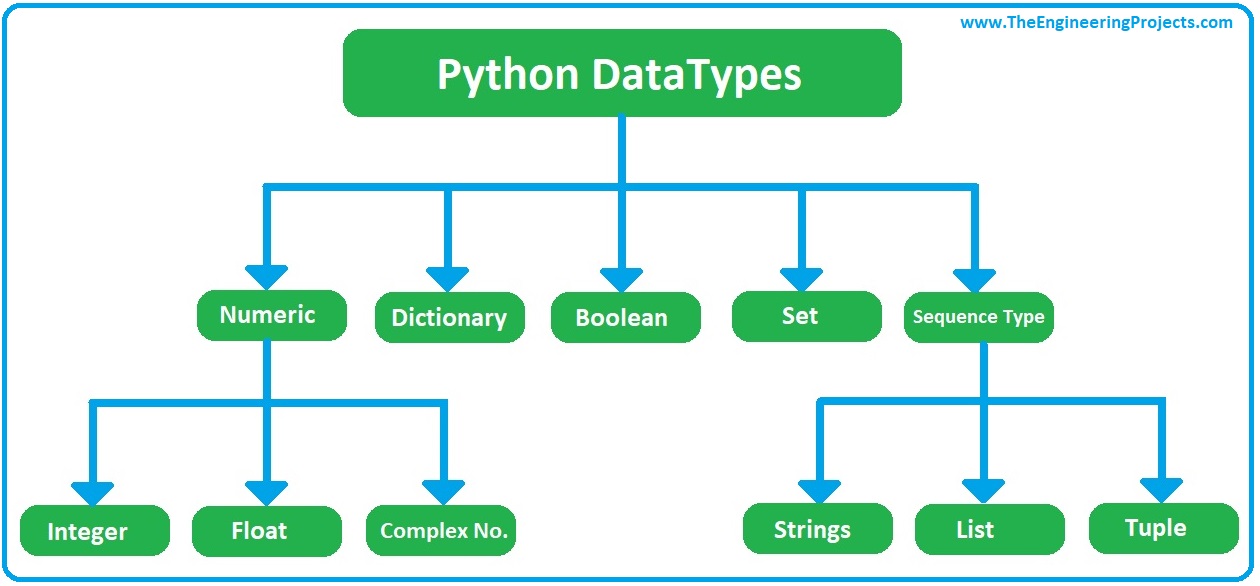
Data types in Python
- Data Types are used for the classification or categorization of similar data packets. There are numerous data types available in python, which we can use depending on our projects' requirement.
- Let's understand data types with an example: Suppose you have some digital data i.e. ON & OFF then you can save this data in Boolean data type but what if your data ranges from 1 to 10, then you need to use integer data types instead of Boolean.
- You can save Boolean data in integer data type but that will be a redundant i.e. we are allocating more space to our data by specifying integer, when we can easily assign Boolean to it.
- Here's the flow chart of available data types in Python language:
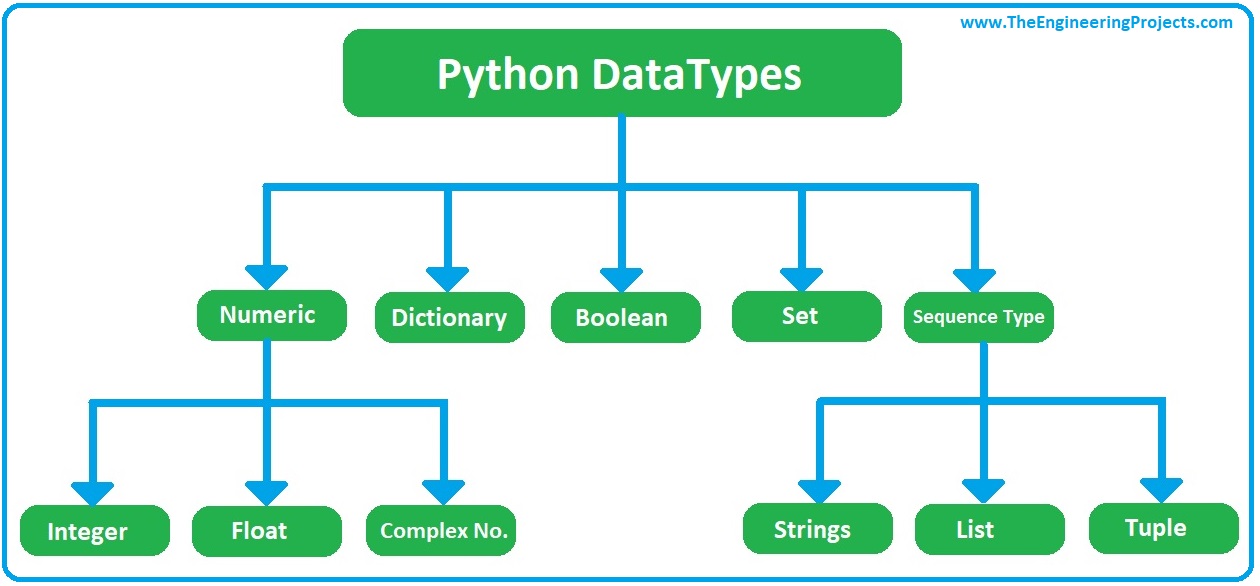
Numeric in Python
- Numeric data types are used to deal with all types of numerical data packets i.e. integer, float etc.
- Numeric data types are further divided into 3 types, which are:
- Integer.
- Float.
- Complex Number.
- Integer (int) data type only holds integer numbers, it could be positive or negative.
- We can't save decimal numbers in integer data type.
- Here's a declaration of a variable x and it is assigned a value a of integer 20:
x = int(20)
Float in Python- Float data types are used to hold decimal numerical values i.e. 2.13, 3.14 etc.
- We can also save whole numbers in float data types.
- Here's a declaration of a variable x and it is assigned a value a of float 20.5:
x = float(20.5)
Complex Numbers in Python- Complex Number data types is used to keep complex numbers in it. Complex numbers are those numerical values which have real & imaginary part.
- That's the versatility of python language, I haven't seen complex number data type in any other programming language.
- Here's a declaration of a variable x and it is assigned a complex number 1+3j:
x = complex(1+3j)
Dictionary in Python
- Dictionary data type is sued to save data in key -> value form. The data is unordered but the value is paired with its key.
- Dictionary data is placed inside curly brackets i.e. {1:"Jones", 2:"IronMan", 3:"Football", 4: "Mosque"}.
- Here's a declaration of a variable x and it's assigned a dictionary data type:
x = dict(name="John", age=36)
Boolean in Python
- Boolean is the simplest data type of all and has just two values assigned to it i.e. True or False.
- Although it's quite simple but its too handy as we have to use it a lot in IF statements. ( We will cover that later )
- Here's a declaration of a variable x, assigned a Boolean data type and it's TRUE:
x = bool(1)
Sequence Type in Python
- Sequence Type data types are used to save data in characters form.
- We can't save numerical data in sequence type but we can convert the two. ( We will discuss that later )
- Sequence Types are further divided into 3 types, which are:
- String.
- List.
- Tuple.
- A string is used to save one or more characters and it's the most commonly used data type.
- Let's understand it with a simple example: You must have seen greeting messages on different projects, we save such data in strings.
- We will discuss strings in detail in our next lecture, where we will perform different operations using strings.
- Here's a declaration of a variable x, which is assigned a string "Hello World":
x = str("Hello World")
List in Python- List data type is used to collect an ordered data, not necessarily of the same type.
- List data is displayed with square brackets.
- We will discuss this in our upcoming lectures in detail, here's a declaration of list:
x = list(("apple", "banana", "cherry"))
Tuple in Python- Tuple data type is used to arrange ordered data, it's quite similar to list but the data is displayed with small brackets.
- Here's a Tuple declaration:
x = tuple(("apple", "banana", "cherry"))
So, we have discussed all these data types in python and if you are not understanding any of them yet then no need to worry as we are going to use them a lot in our coming projects, so you will get them. Before moving to next lecture, let's discuss variables in python a little:Variables in Python
- Variable is a temporary location in computer's memory, which is used to save the data.
- As the name implies, we can change its value using different operations or information given to the program.
- Typically, a program consists of commands that instruct the computer what to do with variables.
- Variables are assigned with tag name, using which we call the value saved in it.
- For examples: x = 5, here x is the name of the variable and 5 is its value.
- In python, we can use special characters, letters, and any number as a variable name.
- Wide spaces and signs with meanings like "+" and "-" are invalid in python.
- You should remember that the names of variables are case sensitive. As the uppercase letter "A" and lowercase letter "a" are considered as different variables.
- As variables are used to save data thus they also assigned a data type. So, a variable could be of int, float or string. (as we seen above)
- In python, it's not necessary to define variable data type, python sets it dynamically.
- There are some variables, which are reserved and we cannot use them.
- We can also change the variables later and assign it to a new variable.
- For example, I have set a value 10 in a variable eat.
eat=100
- Then I added and stored the value of eat+10 in a variable cot.
cot = eat + 10
- So, now cot will be 110.
- X = 456 #integer
- X = 456L #Long integer
- X = 4.56 #double float
- X = "world" #string
- X = [1, 2] #list
- X = (0, 1, 2) #tuple
- X = open('world.py' , 'r') #file
- Variable x, y, and z are assigned with the same memory location with the value of 1.
x = y = z = 1
- Let's create few variables in python, I have created first_name, date_of_birth & last_name, as shown in below figure:
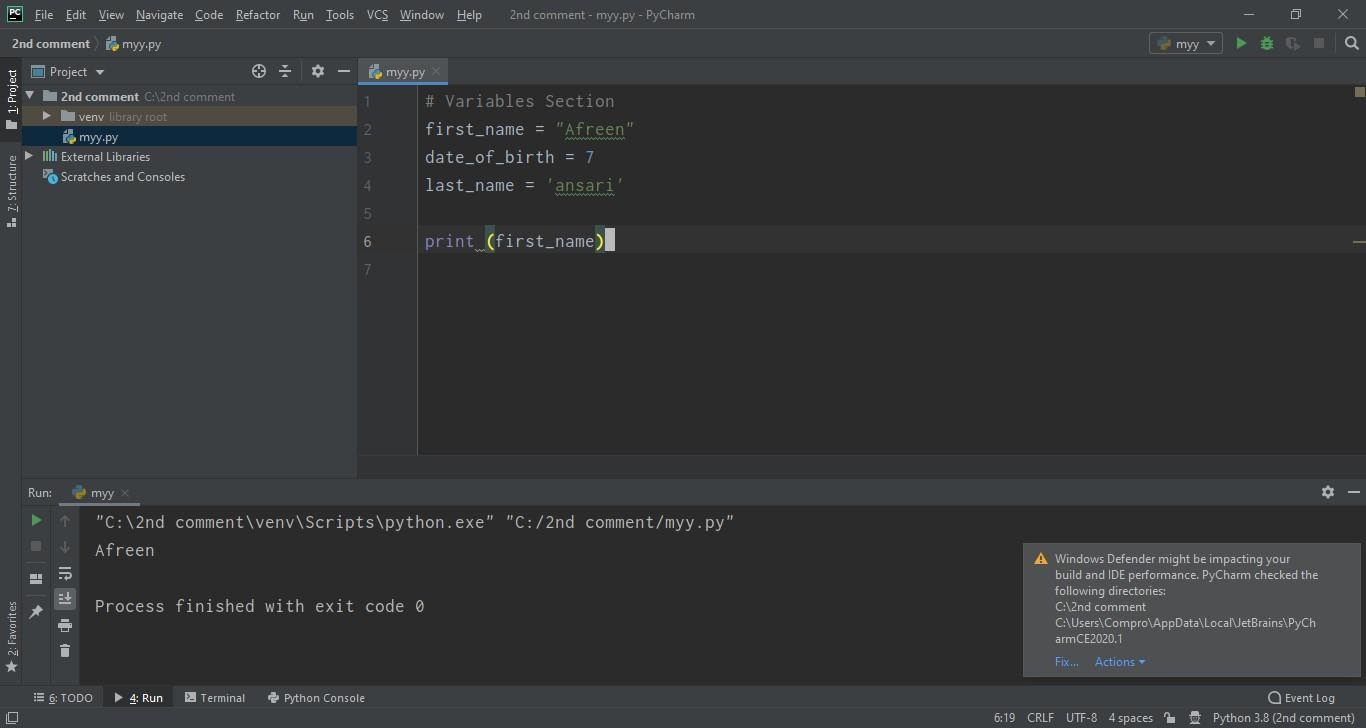
- I have printed first_name and its appeared in the output panel.