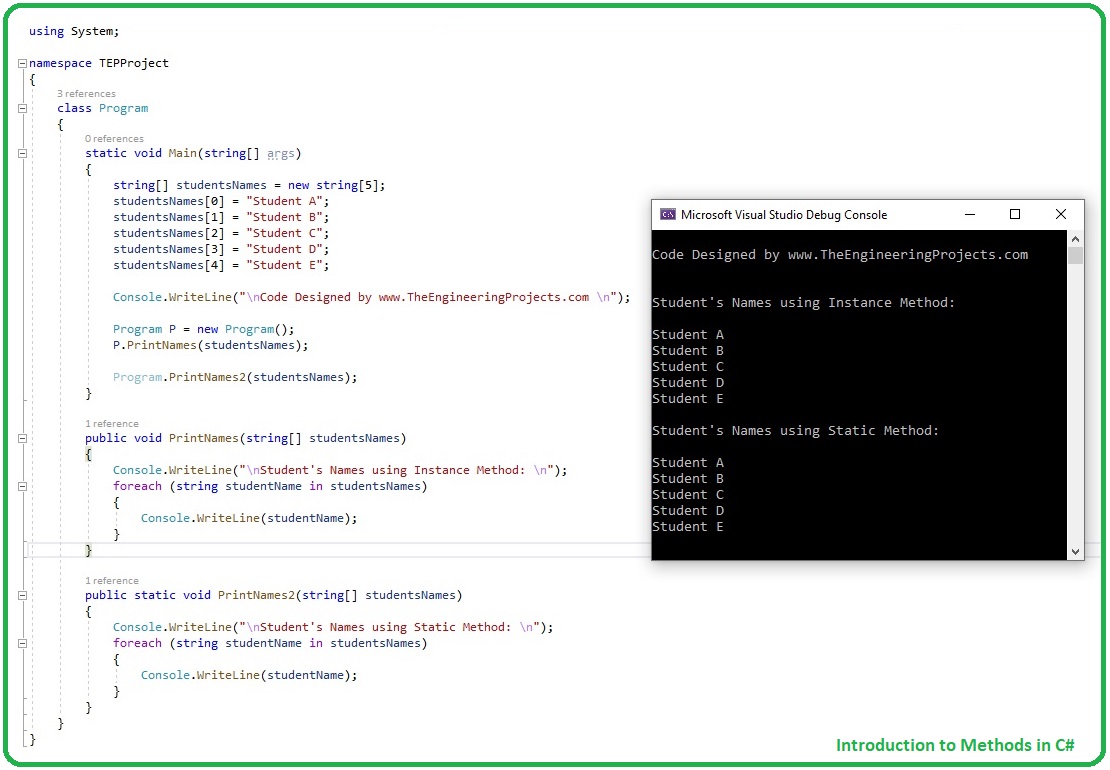
Introduction to Methods in C#
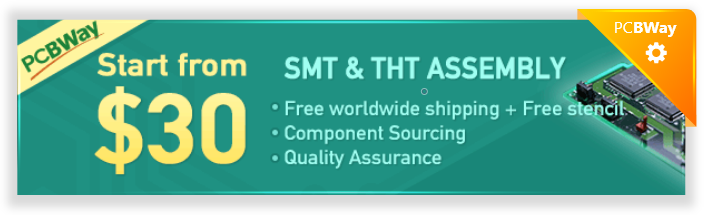
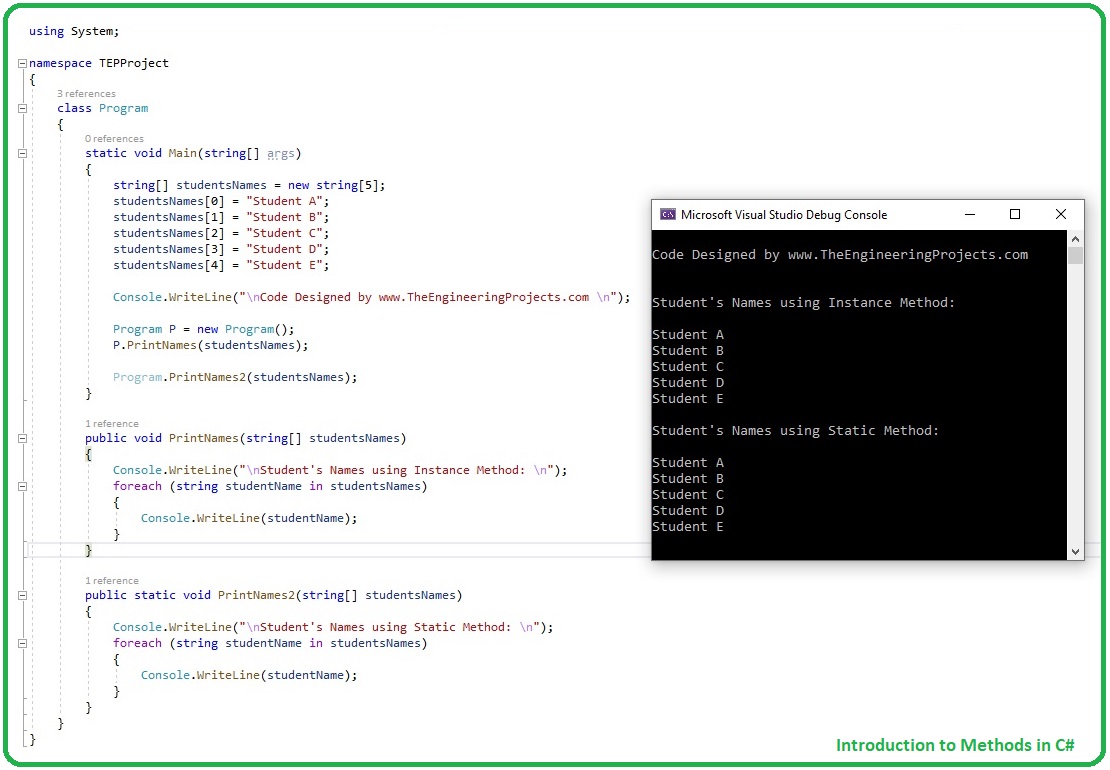
Introduction to Methods in C#
- Methods in C#, also called Functions, are extremely useful in optimizing the code, normally used to create a logic once and use it repeatedly.
- It happens in projects where you need to do a similar job at various places of your code.
- In such conditions, it's wise to create a small function of your repeated code and instead of adding those lines again and again, simply call the function.
- Here's the syntax of Methods in C#:
Access-Modifiers Return-Type Method-Name ( Parameters ) { // Method-Body } public void HelloWorld(){ Console.Write("Hello World !!!"); }
- Access-Modifiers: Access Modifiers are used to control access capability of any method / function. You can set them public, protected or private etc. We will discuss them later in detail.
- Return-Type: It decides what the method is gonna return, it could be void or any data type i.e. int, float, string etc.
- Method-Name: It's the unique name of the Method / Function, which can't be any reserved keywords in C#. It's should be meaningful so you can remember later.
- Parameters: Parameters are optional and are normally used to transfer data between methods.
- There are two types of methods available in C#, which are:
- Instance Method.
- Static Method.
- Let's discuss both of them separately, in detail:
Instance Method in C#
- Those methods, which doesn't have static keyword in their definition are called Instance Methods in C#.
- In order to call instance method in another method, we have to first create a new instance of that method and the invoke the method using dot operator.
- Let's have a look at how to create and call an instance method in C#:
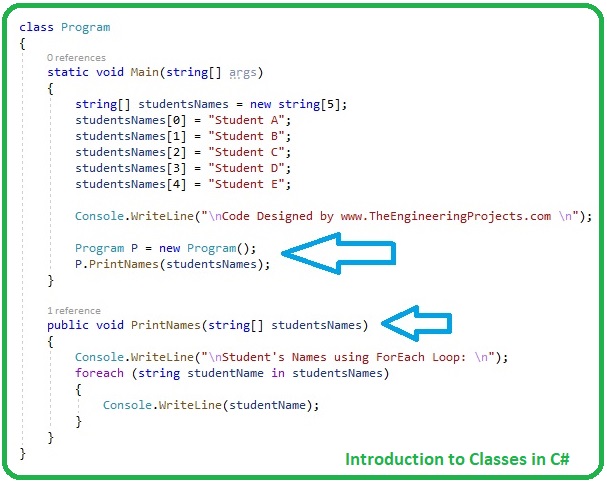
- You must have realized by now that all the work we have been doing so far was in Main Method. That's the default method, the C# compiler first goes into and it's a static method as it has static keyword in it, which will discuss next.
- So, now in above figure, I have created a new method and I have used public (access-modifier) and void (return-type) and the Method-Name is PrintNames. I am using studentsNames array as a parameter.
- I have used the same code which we have designed in our previous lecture on For Loop in C#, so I have placed the foreach loop in my newly created instance method named PrintNames.
- Now in order to call this new method in Main method, I have to first create a new instance of this method's class and you must have noticed that the class name is Program at the top.
- Both of our Main & PrintNames Methods are placed inside Program class.
- So, in our Main function, I have first created a new instance of my class Program using new keyword:
Program P = new Program ( );
- After that, using this class instance P, I have invoked the PrintNames method using dot operator ( . ).
P.PrintNames ( studentsNames ) ;
- When you are calling your method, make sure you enter the parameters correctly as specified in its definition otherwise compiler will generate an error.
- You can specify multiple parameters separated by commas.
- So, that's how we can create and call an instance method, so now let's have a look at How to call static method in C#.
Static Method in C#
- Those methods, which have static keyword in its definition are called static methods in C#.
- In order to call a static method, we don't need to create a new instance of Program class.
- Instead, we can directly invoke a static method from Program class using dot operator ( . ), as shown in below figure:
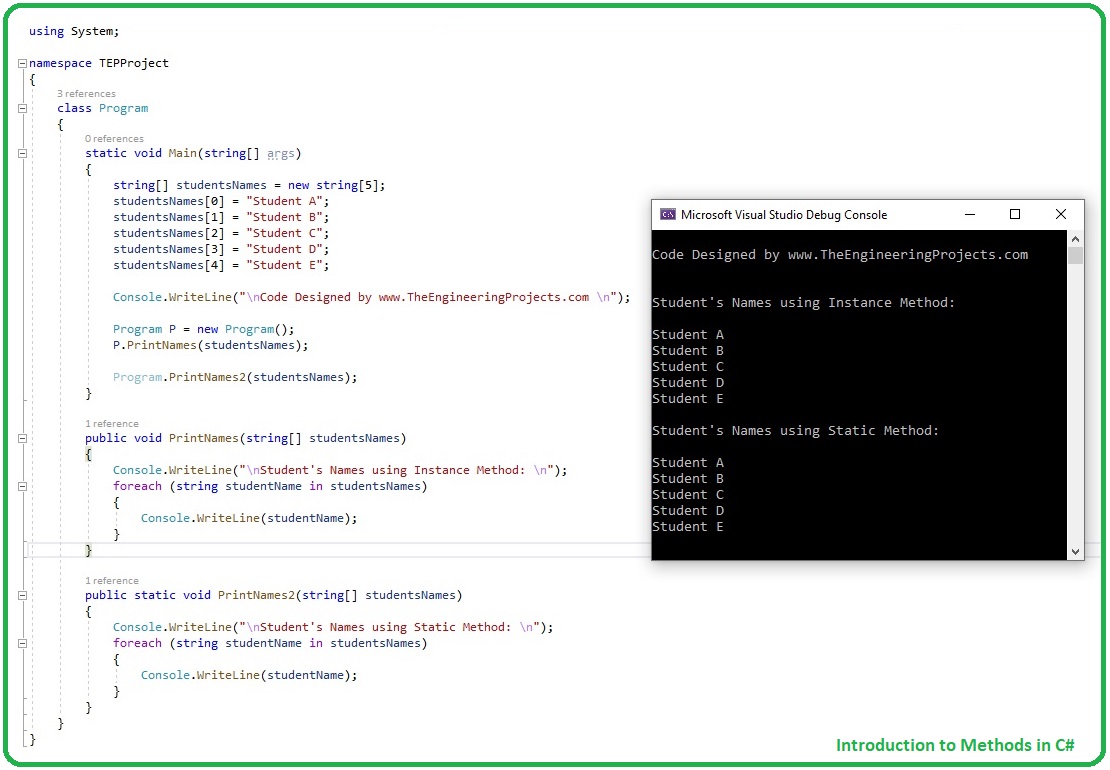
- In above figure, I have created a new method called PrintNames2 and I have used static keyword in its definition, so its a static method in C#.
- So, now in order to call that method, I have used the name of class and then invoked the method using dot operator, using below code:
Program.PrintNames2 ( stringNames );
- We don't need to create a new instance of class for static method, that's the difference between static and instance methods in C#.
×
![]()