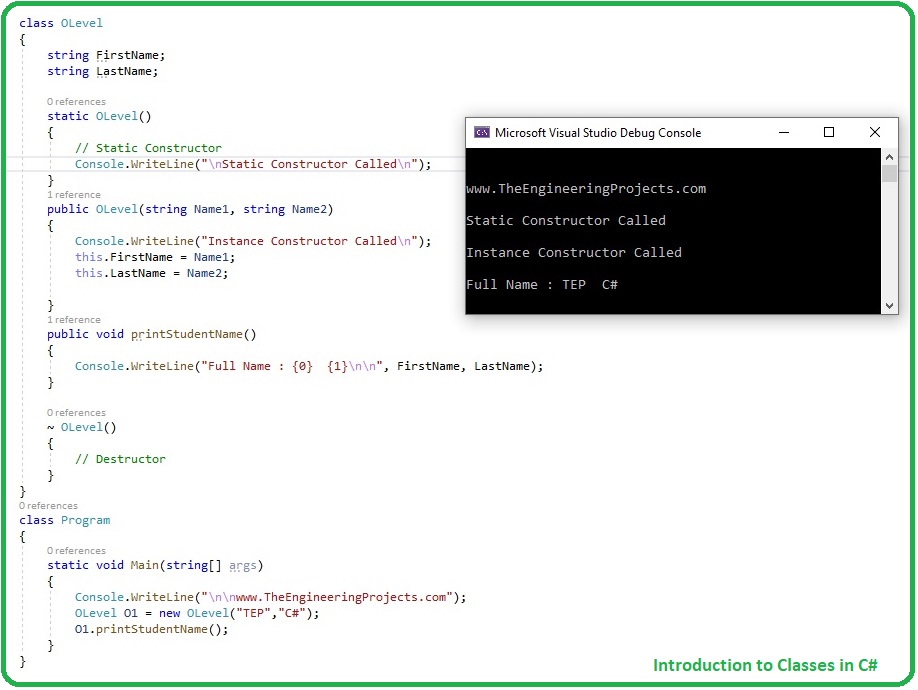
Introduction to Classes in C#
- Classes in C# are referred as storage units for different methods, fields, objects etc. and are used for organizing the code.
- In our previous lessons in C#, we have always taken an example of a classroom but what if we have to create a software for an entire school system.
- In that case, we can create separate teams, dealing with each classroom, so you can think of that classroom as a class in C#.
- Our Main method is in class Program, Let's create a new class for OLevel classroom students:
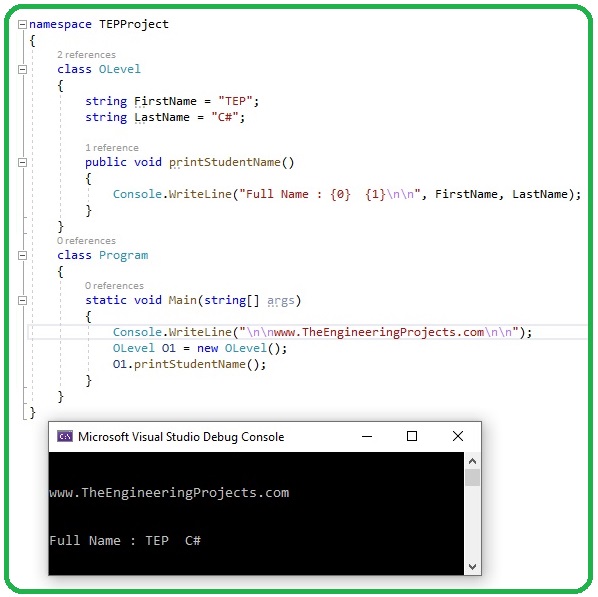
- so, in the above figure, you can see that I have created a new class named OLevel and this class has 3 members, named as:
- First one is field/variable named: FirstName.
- Second one is field/variable named: SecondName.
- Third one is method named: printStudentName.
- So, we have 3 members in our newly created class and all these members are instance members as they don't have static keyword in their statement.
- So, in order to call this OLevel method in Main method, we have created a new instance of OLevel class, as we did in our previous lecture on methods in C#.
- After that using this new instance of OLevel class, we have invoked the printStudentName method using dot operator.
- When we run our code, we have the Full Name : TEP C#, as given in the code.
- You can also use Constructors & Destructors in C#, but they are not necessary to use, as we haven't used the constructor but our code worked fine.
- So, let's have a look at what are these terms one by one:
C# Class Constructors
- C# Class Constructor is a simple method / function, which gets executed automatically whenever the new instance of class is created.
- It must have the same name as the class itself and it won't have a return type and the access modifier is public.
- Constructors are normally used for initializing data fields or for initial settings of your class methods.
- Let's create a Constructor for our OLevel class:
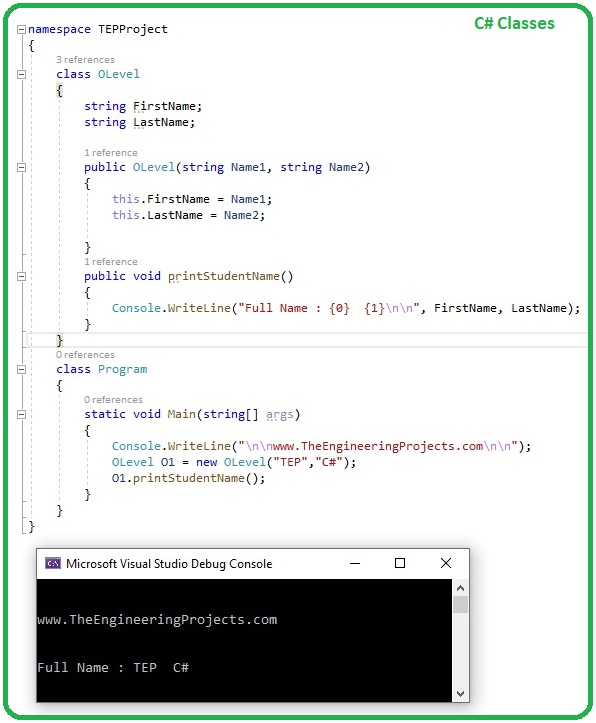
- In above figure, you can see I have created a new method in our OLevel class and it has the same name OLevel.
- Constructors can also have parameters, so if we want to send some data from one class to another class, we can use these constructor parameters.
- So, now instead of hard coding the data in my new class, I am sending the data, when I am create new instance of class. i.e.
OLevel O1 = new OLevel ( " TEP " , " C# " );
- So, that way I can send multiple data just by creating new instance of class.
- You must have noticed that this C# Class Constructor doesn't have a static keyword in it so its an Instance Constructor.
- So, we can also add a static Constructor in C# class, which don't have any access modifier or return type and just have static keyword in its definition and is executed before instance Constructor.
- Moreover, Destructor in C# is used to clean up any resources used by the class and these destructors are called automatically by the garbage collector so we don't need to worry about them.
- Destructors can't take any parameters and they doesn't have access modifier or return type, they just have a tilled sign ( ~ ) in front of them.
- I have created static Constructor, Instance Constructor & Destructor in below code:
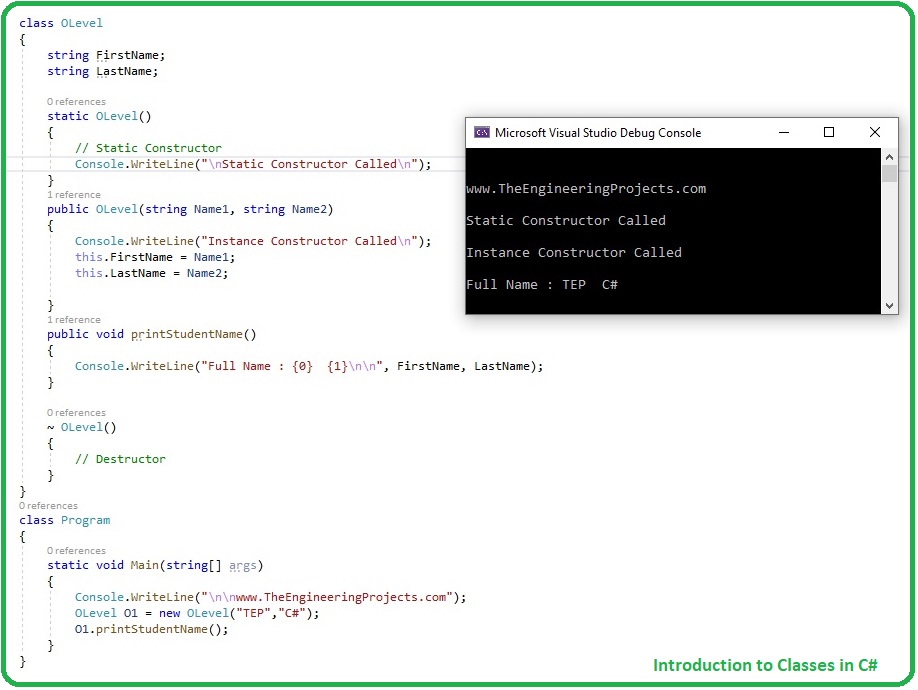
- You can see in above figure, that static Constructor is called first and then instance Constructor is called and finally we printed the Full Name.
- Here's the complete code used in this lecture:
using System; namespace TEPProject { class OLevel { string FirstName; string LastName; static OLevel() { // Static Constructor Console.WriteLine("\nStatic Constructor Called\n"); } public OLevel(string Name1, string Name2) { Console.WriteLine("Instance Constructor Called\n"); this.FirstName = Name1; this.LastName = Name2; } public void printStudentName() { Console.WriteLine("Full Name : {0} {1}\n\n", FirstName, LastName); } ~ OLevel() { // Destructor } } class Program { static void Main(string[] args) { Console.WriteLine("\n\nwww.TheEngineeringProjects.com"); OLevel O1 = new OLevel("TEP","C#"); O1.printStudentName(); } } }So, that was all about Classes in C#, I hope you have understand their basic concept, we will gradually move towards complex codes. In the next session, we will have a look at Introduction to Namespaces in C#. Till then take care & have fun !!! :)