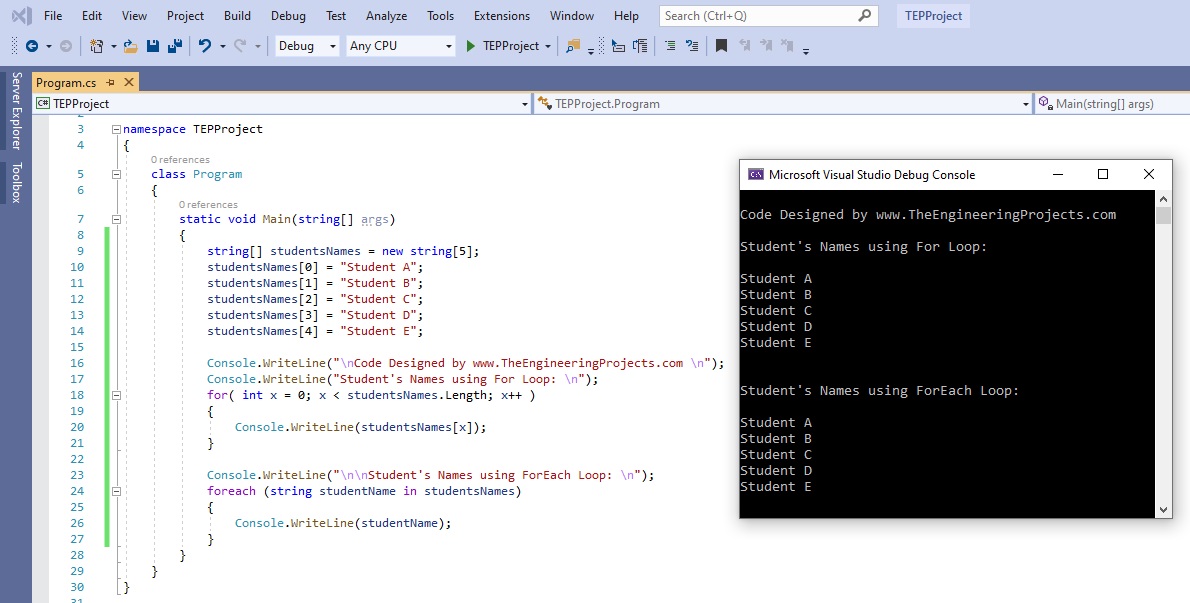
How to use for Loop in C#
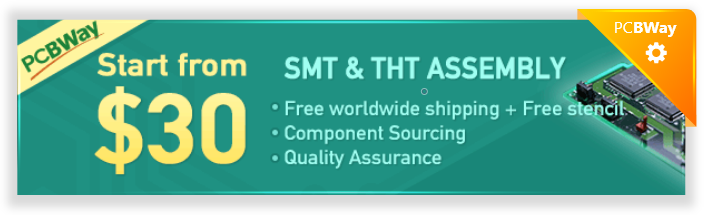
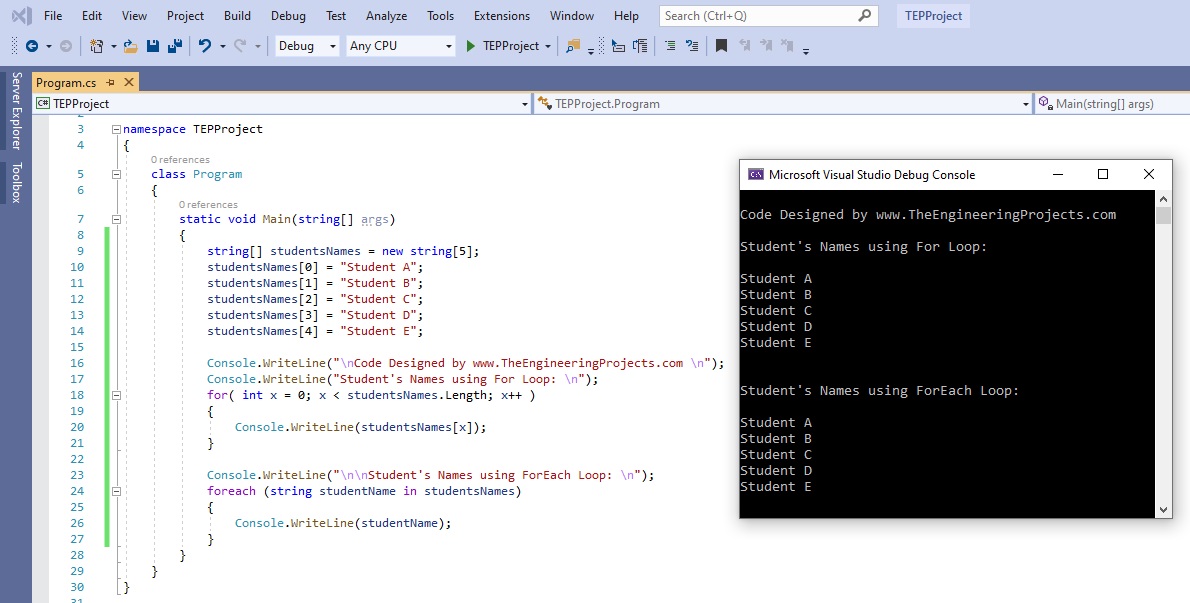
How to use for Loop in C#
- For loop in C# takes an integer variable as a Controlling agent, initialized at value V1 and ends at value V2, and the travel from value V1 to V2 depends on the Condition specified in () brackets.
- In both IF loop & while loop, the small brackets just have single argument, but in for loop, the small brackets have 3 arguments, separated by semicolon ; , which are:
- First argument is initial value of variable V1.
- Second argument is final value of variable V2.
- Third argument is the condition applies on this variable i.e. increment, decrement etc.
- The loop will keep on repeating itself and we will also have the value of iteration in the form of variable value.
- Let's have a look at its syntax:
for (Initial Value V1; Final Value V2; Condition) { // body of for loop }
- Now let's have a look at a simple for loop in action
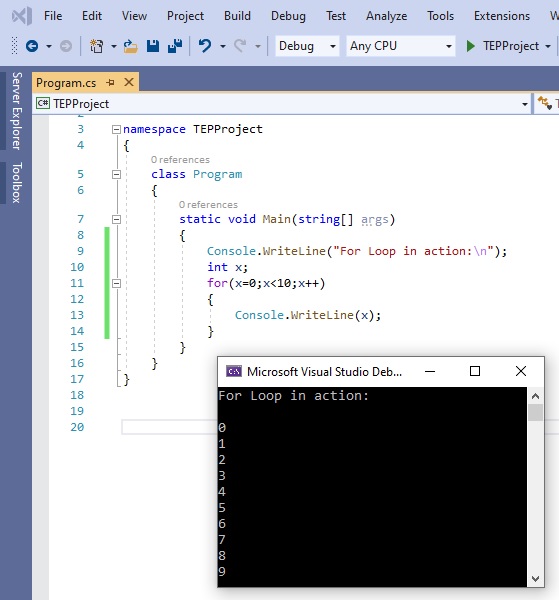
- Now you can see in above figure that I have initialized an integer variable x and in for loop, I have first assigned the initial value x=0 in first argument.
- In second argument, separated by ; , I have specified the final value x<10.
- Finally in third argument, I have incremented the variable x++.
- So, now when the compiler will first reach for loop, it will get x=0 and will run the code inside { } brackets that's why when I printed the value of x, it was 0 at first.
- After running all the lines in { } brackets, compiler will run the condition, which is to increment the variable, so in second iteration, the value of x=1, that's why in second line we have 1 on console.
- So, this loop will keep on running and the variable will keep on incrementing and when it will reach x=9, it will run the code lines in { } brackets and at the end it will increment the variable and will make it x = 10.
- So, now at x=10, the compiler knows in second argument of For loop, the variable's last value is 9 i.e. x <10. So when the value is not x<10, the compiler will leave for loop and go to next line below for loop.
- So, that's how For loop works, we can use it for running some lines of code repeatedly and the use of this variable inside for loop is quite helpful.
- Here's an example, where I have decremented the variable in third argument:
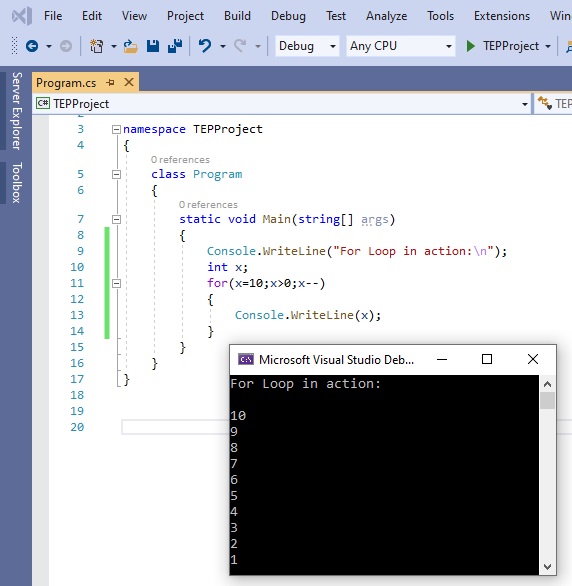
- From the console, its quite evident that now the variable's value is decreasing from 10 to 1, I have used x - - in condition part of For Loop.
- Now let's create an array of students' names and display all elements of that array using for Loop, we have discussed How to use Arrays in C# in our 6th lecture, you should recall that as well.
- Here's the code and it's output on console:
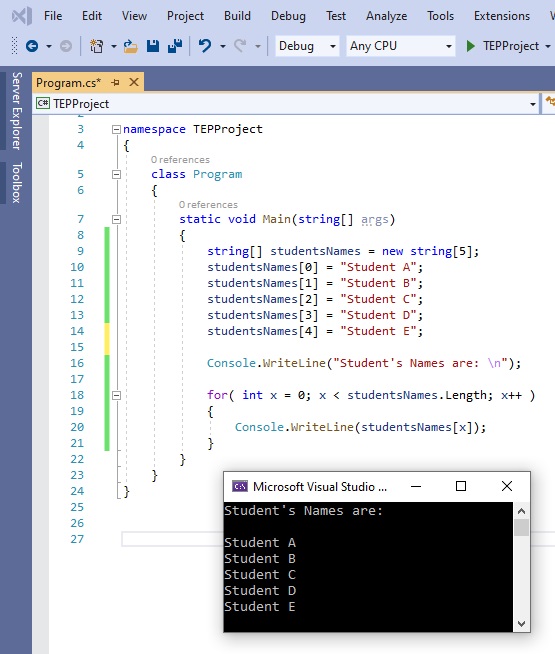
- As you can see in above figure that first I have initialized a new String Array of size 5.
- After that added some data i.e. students names, in each element of C# array.
- Next, I have used for Loop and initialized the variable and also assigned the first value 0 in first argument.
- In the second argument, I have used a Length property of array and our array's length is 5.
- So, this for loop will run from 0 to 4 and we can see at the console output that it has printed all the elements of array i.e. students names.
- That's how, we can use for Loop in C#, now let's have a look at how to use foreach loop in C#, which is kind of an extension of for loop.
How to use Foreach Loop in C#
- We have discussed Foreach Loop in our 6th tutorial on arrays and I have told you that we will discuss it in detail later, so now is the time to discuss it out.
- Foreach loop in C# is used to iterate through a collection or arrays from start till end. Collections could be ArrayList, HashTable etc. we will discuss them later.
- Let's first have a look at its syntax:
foreach (item in collections/arrays) { // body of foreach loop }
- This item variable will go through the whole array and will repeat the lines of code inside { } brackets.
- So, let's rewrite our previous example with foreach loop along with for loop and look at both results:
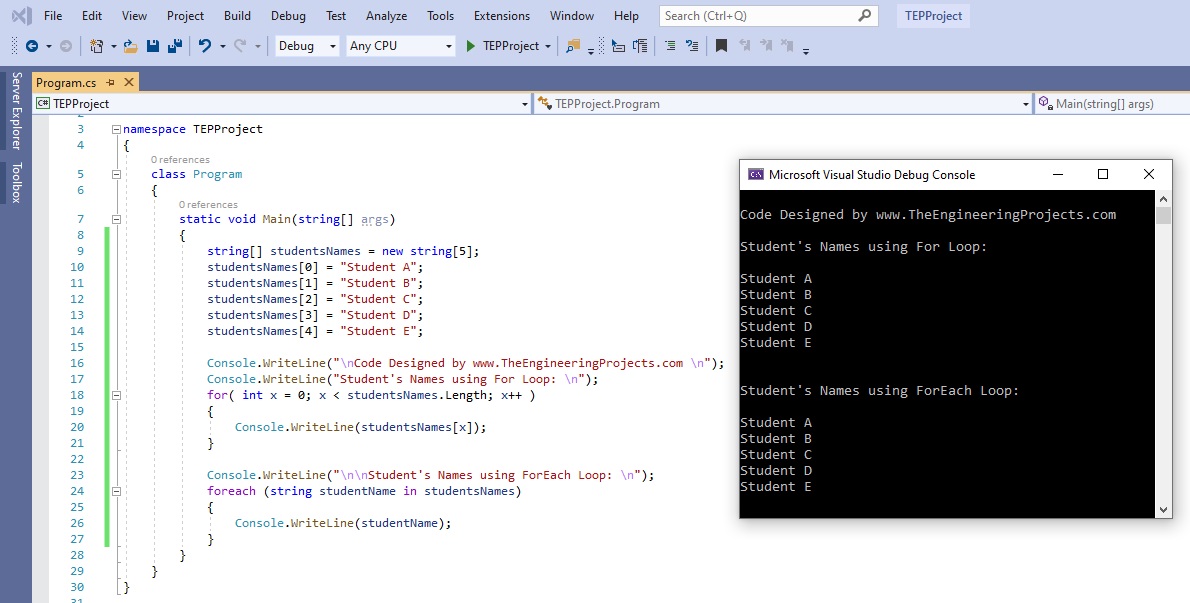
- Now you can see in above figure that we got similar results for both loops although foreach loop is quite simple and easy to look at thus reduces your code.
- In foreach loop, we are directly accessing the elements of array, while in for loop, we are getting elements using index of array.
×
![]()