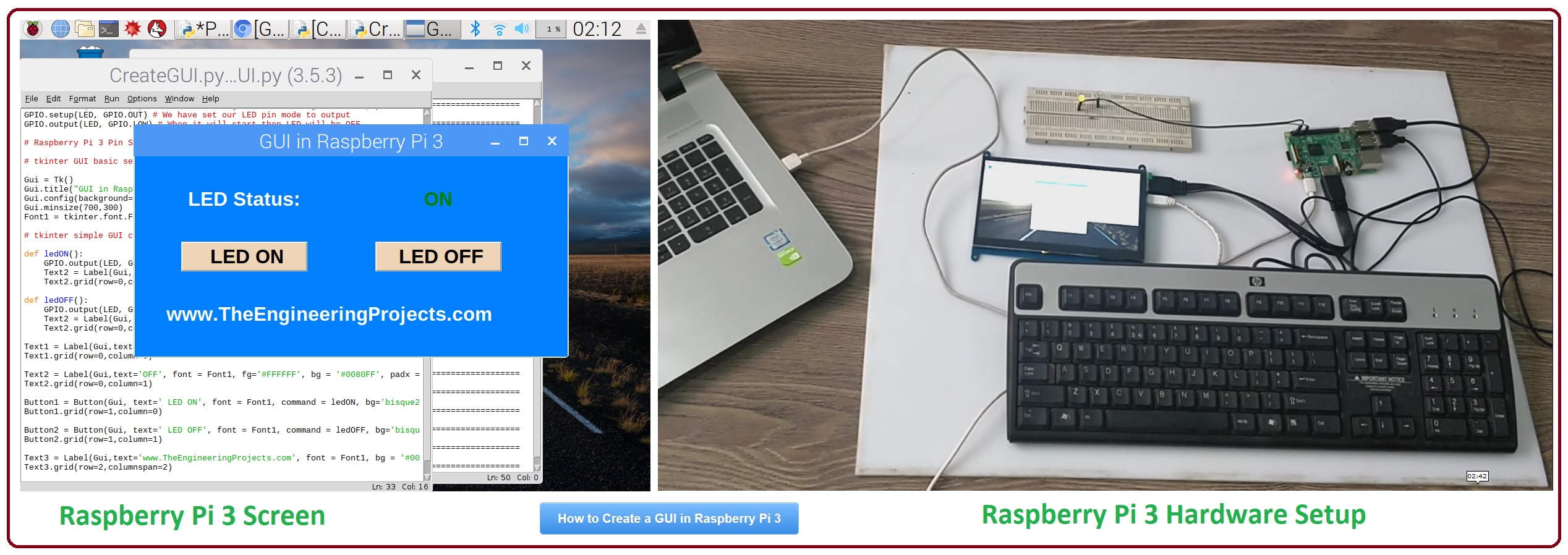
How to Create a GUI in Raspberry Pi 3
- I hope you have already Setup your HDMI LCD with Raspberry Pi 3, as we have done in our previous tutorials.
- Here's an image of our final setup:
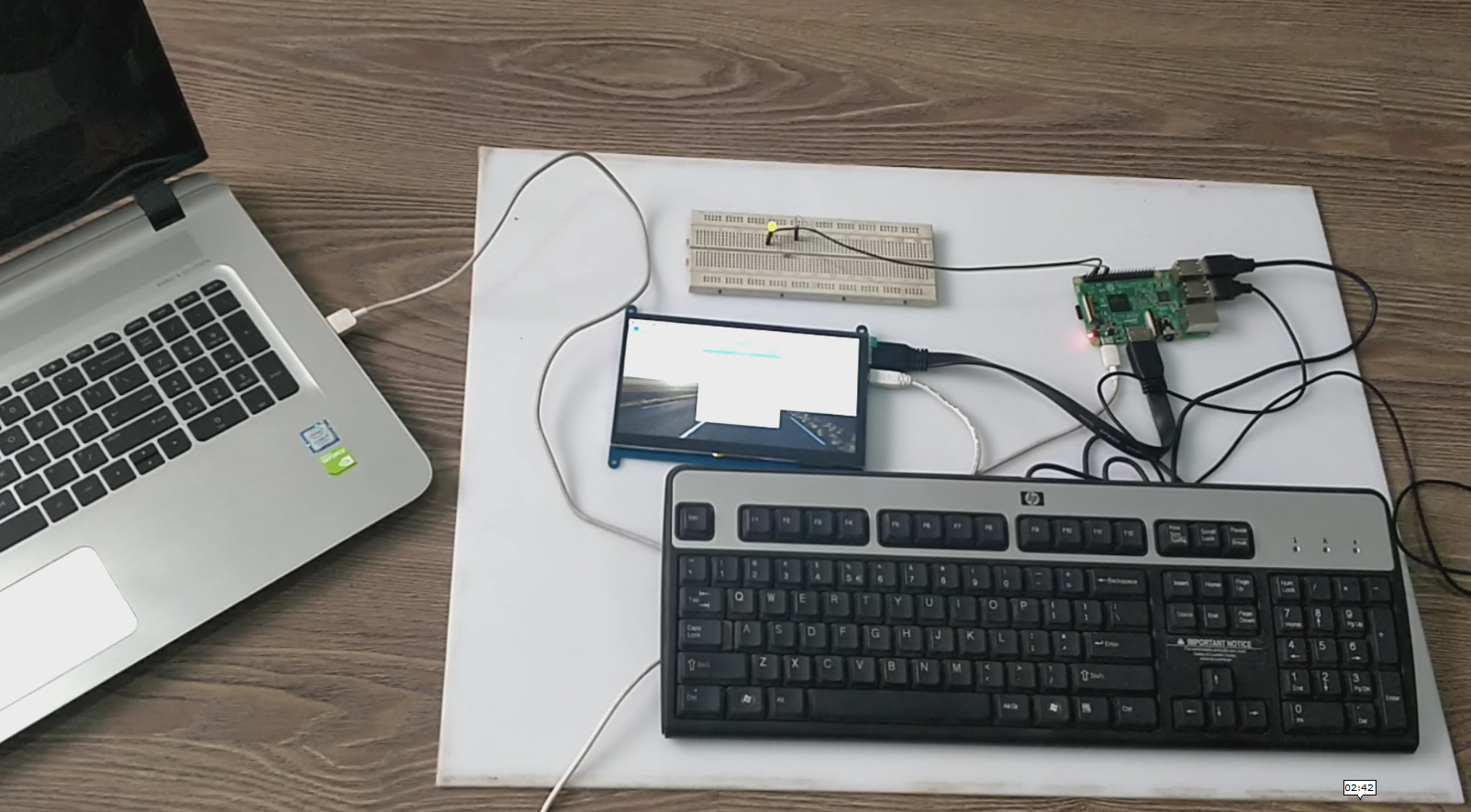
- So open a new File in your Python IDLE and save it, I have given it a name CreateGUI.py
- First of all we are gonna import our libraries in python, so here's the code:
# ************************************************************************** # # **** **** # # *********** Code Designed by www.TheEngineeringProjects.com ************** # # **** **** # # ****************** How to Create a GUI in Raspberry Pi 3 ***************** # # **** **** # # ************************************************************************** # # Importing Libraries import RPi.GPIO as GPIO import time from tkinter import * import tkinter.font # Libraries Imported successfully
- In the above code you can see, we have imported a new library which is tkinter library and we have also imported font from it.
- Now we are gonna do some initial settings of our Raspberry Pi 3 LED Pin, as we have have done in LED Blinking using Raspberry Pi 3.
- Here's the code for that:
# Raspberry Pi 3 Pin Settings LED = 11 # pin11 GPIO.setwarnings(False) GPIO.setmode(GPIO.BOARD) # We are accessing GPIOs according to their physical location GPIO.setup(LED, GPIO.OUT) # We have set our LED pin mode to output GPIO.output(LED, GPIO.LOW) # When it will start then LED will be OFF # Raspberry Pi 3 Pin Settings
- In the above code I have set all warnings to False as I think warnings are just annoying. :P
- After that I have set our GPIO mode to Board because its lot easier to remember Pin Number as their number on board.
- You can set this mode to BCM as well.
- Next we have made our LED Pin output and have made it Low as I wanna keep my LED in OFF state when project starts up.
- Now let's place some code for GUI initialization and basic settings,here's the code:
# tkinter GUI basic settings Gui = Tk() Gui.title("GUI in Raspberry Pi 3") Gui.config(background= "#0080FF") Gui.minsize(700,300) Font1 = tkinter.font.Font(family = 'Helvetica', size = 24, weight = 'bold') # tkinter simple GUI created
- First of all, I have create an object named Gui, which is actually my GUI Board.
- Then I have given it a title, which will appear as name of this GUI or software.
- I have made the background light blue, it will look good.
- the minimum size, I have set is 700 x 300, its in pixels (x, y).
- Finally we have set our Font, which we are not using yet but will use in next section.
- Now if you run your code then you will get something as shown in below figure:
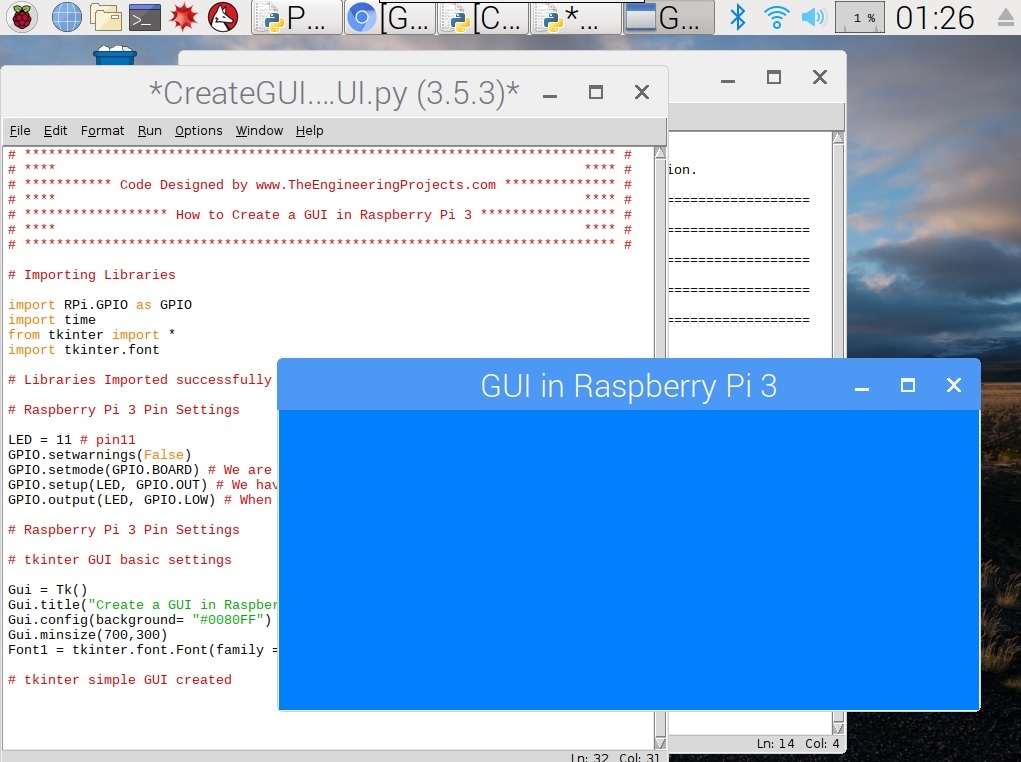
- You can see in above figure that we a got a simple board and the title is also there.
- So, now let's add some Labels first on our GUI in pi 3:
Text1 = Label(Gui,text='LED Status:', font = Font1, fg='#FFFFFF', bg = '#0080FF', padx = 50, pady = 50) Text1.grid(row=0,column=0) Text2 = Label(Gui,text='OFF', font = Font1, fg='#FFFFFF', bg = '#0080FF', padx = 0) Text2.grid(row=0,column=1)
- I think these codes are self explanatory, I have added two texts "LED Status: " and "OFF".
- I have given them the Font1 which we created in last section and then the background is again white, and I have also added some padding in x and y direction so that it won't touch the borders.
- In this GUI tkinter coding we have grid system. The below image will clear the idea:
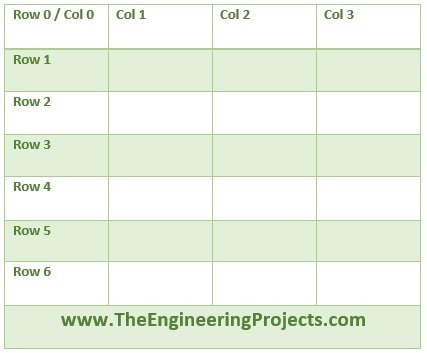
- We have added both our Labels in first row, so here's the output of above code:
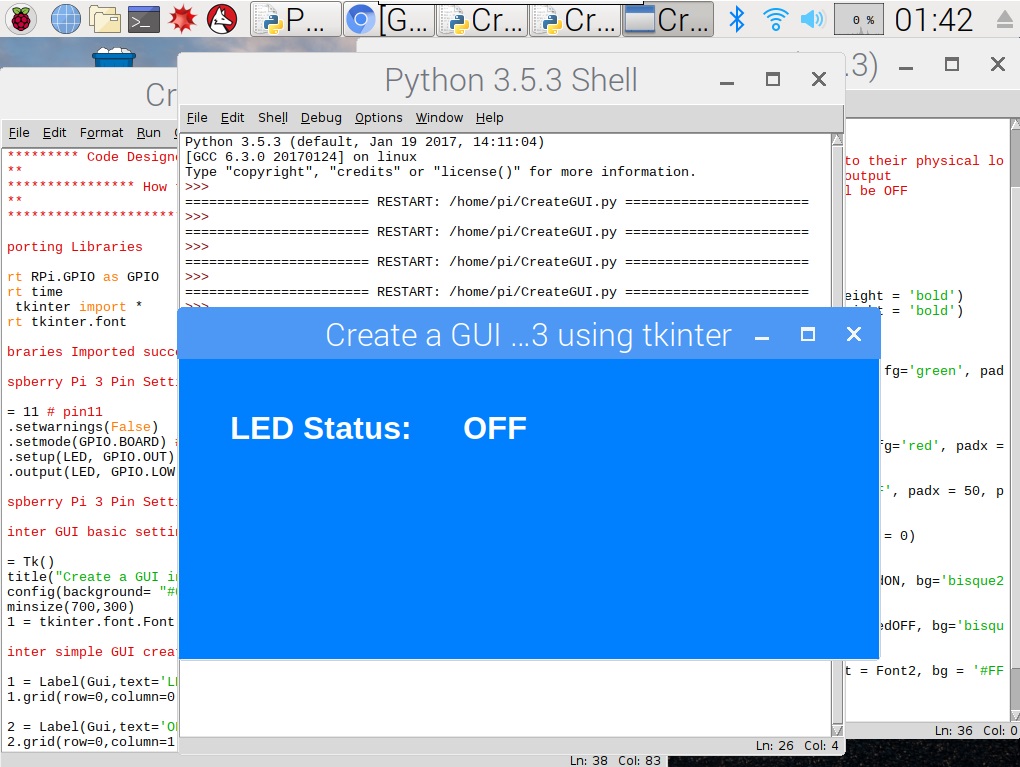
- Now let's add two buttons below these Labels, which will control our LED and will turn it ON and OFF.
- Here's the code for adding Buttons in GUI using tkinter:
Button1 = Button(Gui, text=' LED ON', font = Font1, command = ledON, bg='bisque2', height = 1, width = 10) Button1.grid(row=1,column=0) Button2 = Button(Gui, text=' LED OFF', font = Font1, command = ledOFF, bg='bisque2', height = 1, width = 10) Button2.grid(row=1,column=1)
- You have seen in above code that now these two buttons are in second row so they will come below these Labels.
- The only thing worth mentioning here is the Command, its actually a function which will execute on pressing that button.
- So, now we need to add these functions above Label codes.
- Just try to understand the code rite now, I have shared the complete file below, which you can easily download.
- So, here's these two functions' codes:
# Function for Buttons started here def ledON(): GPIO.output(LED, GPIO.HIGH) # led on Text2 = Label(Gui,text=' ON ', font = Font1, bg = '#0080FF', fg='green', padx = 0) Text2.grid(row=0,column=1) def ledOFF(): GPIO.output(LED, GPIO.LOW) # led off Text2 = Label(Gui,text='OFF', font = Font1, bg = '#0080FF', fg='red', padx = 0) Text2.grid(row=0,column=1) # Function for Buttons ended here
- In some cases you need to merge your columns or rows, for that you can use below code.
- In below code, I have merged the columns of last row by using 'columnspan=2' and added our site's link.
- It has merged the first two columns of last row and here's the code:
Text3 = Label(Gui,text='www.TheEngineeringProjects.com', font = Font1, bg = '#0080FF', fg='#FFFFFF', padx = 50, pady = 50) Text3.grid(row=2,columnspan=2)
- And finally we need to add our main loop code, which is like while(1) in python, it is given below:
Gui.mainloop()
- Now run your code and you will get something as shown in below figure:
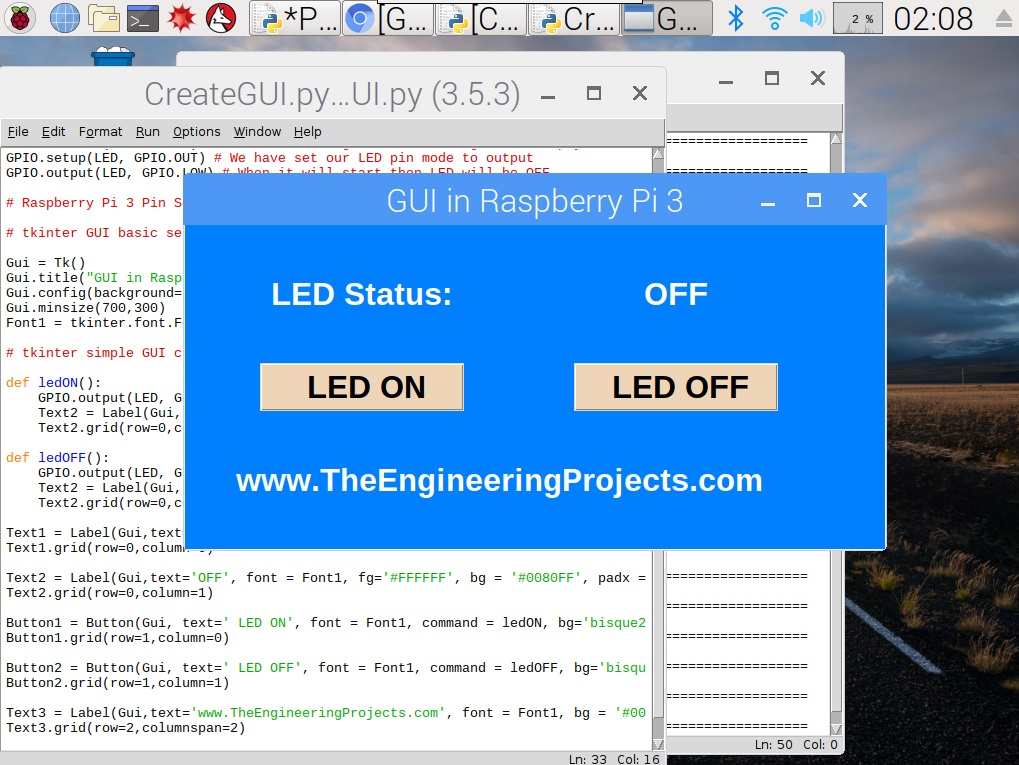
- I have combined all the above codes and here's it's final form, it's now easy to understand:
# ************************************************************************** # # **** **** # # *********** Code Designed by www.TheEngineeringProjects.com ************** # # **** **** # # ****************** How to Create a GUI in Raspberry Pi 3 ***************** # # **** **** # # ************************************************************************** # # Importing Libraries import RPi.GPIO as GPIO import time from tkinter import * import tkinter.font # Libraries Imported successfully # Raspberry Pi 3 Pin Settings LED = 11 # pin11 GPIO.setwarnings(False) GPIO.setmode(GPIO.BOARD) # We are accessing GPIOs according to their physical location GPIO.setup(LED, GPIO.OUT) # We have set our LED pin mode to output GPIO.output(LED, GPIO.LOW) # When it will start then LED will be OFF # Raspberry Pi 3 Pin Settings # tkinter GUI basic settings Gui = Tk() Gui.title("GUI in Raspberry Pi 3") Gui.config(background= "#0080FF") Gui.minsize(700,300) Font1 = tkinter.font.Font(family = 'Helvetica', size = 24, weight = 'bold') # tkinter simple GUI created # Funtion for Buttons started here def ledON(): GPIO.output(LED, GPIO.HIGH) # led on Text2 = Label(Gui,text=' ON ', font = Font1, bg = '#0080FF', fg='green', padx = 0) Text2.grid(row=0,column=1) def ledOFF(): GPIO.output(LED, GPIO.LOW) # led off Text2 = Label(Gui,text='OFF', font = Font1, bg = '#0080FF', fg='red', padx = 0) Text2.grid(row=0,column=1) # Funtion for Buttons ended here Text1 = Label(Gui,text='LED Status:', font = Font1, fg='#FFFFFF', bg = '#0080FF', padx = 50, pady = 50) Text1.grid(row=0,column=0) Text2 = Label(Gui,text='OFF', font = Font1, fg='#FFFFFF', bg = '#0080FF', padx = 0) Text2.grid(row=0,column=1) Button1 = Button(Gui, text=' LED ON', font = Font1, command = ledON, bg='bisque2', height = 1, width = 10) Button1.grid(row=1,column=0) Button2 = Button(Gui, text=' LED OFF', font = Font1, command = ledOFF, bg='bisque2', height = 1, width = 10) Button2.grid(row=1,column=1) Text3 = Label(Gui,text='www.TheEngineeringProjects.com', font = Font1, bg = '#0080FF', fg='#FFFFFF', padx = 50, pady = 50) Text3.grid(row=2,columnspan=2) Gui.mainloop()
- If you got into any trouble then ask in comments.
- Now when you click on the "LED ON" Button then your LED will turn ON and when you click on the "LED OFF" button, then your LED will go OFF.
- LED status text on GUI will change from OFF to ON.
- Both Raspberry Pi 3 Screen and its hardware setup are shown in below figure: (click & zoom)
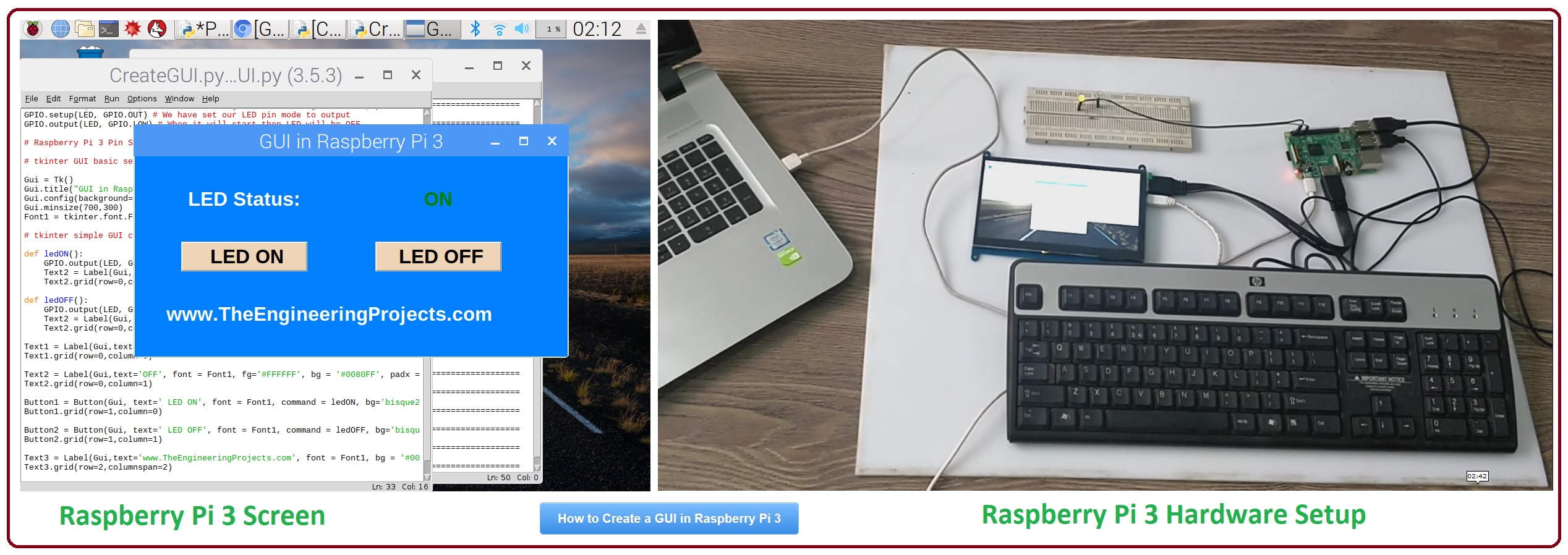
- Now when you click the LED OFF button then LED status will change from ON to OFF as shown in below figure:
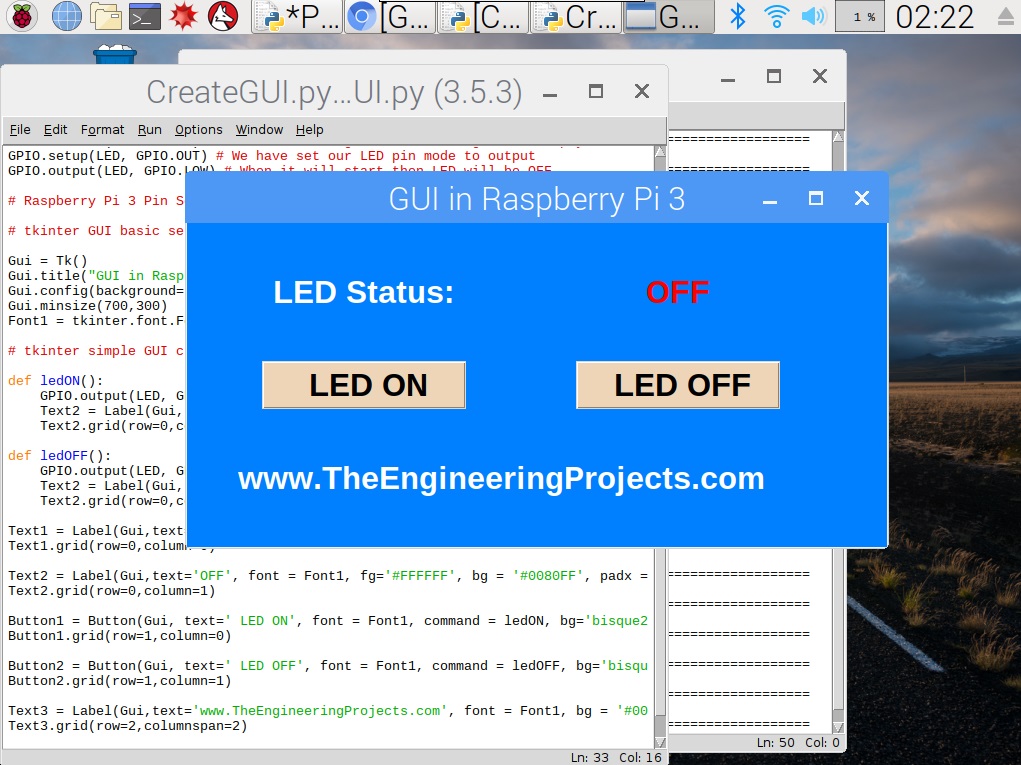
- I hope you have got the main idea of How to create GUI in Raspberry Pi 3.
- In my coming tutorial, I will share many tutorials on raspberry Pi 3 in which we will create such simple GUIs and will display different sensors' values and will also control them.
- You can download this CreateGUI.py file by clicking the below button:
[dt_default_button link="https://www.theengineeringprojects.com/RaspberryPi3/CreateGUI.rar" button_alignment="default" animation="fadeIn" size="medium" default_btn_bg_color="" bg_hover_color="" text_color="" text_hover_color="" icon="fa fa-chevron-circle-right" icon_align="left"]Download CreateGUI.py File[/dt_default_button]
So, that was all about How to Create GUI in Raspberry Pi 3. I hope you have got the detailed idea of GUI creation. In my coming tutorial, we will have a look at How to Control DC Motor with Raspberry Pi 3. Thanks for reading, have fun !!! :)