While today we will have a look at how to send the data through a serial port in Arduino and for that, I am going to use the Arduino Serial Write command. It's also going to be a very simple and basic Arduino tutorial but if you are new to Arduino then you must read it completely as it will gonna help you out. I have also designed a Proteus Simulation and explained it at the end of this tutorial. I hope you guys are gonna learn from it:
How to use Arduino Serial Write ???
- For the basics on Serial Port, you should read What is Serial Port?
- And as I have explained in my previous tutorial How to use Arduino Serial Read that Arduino has a Serial Port at its Pin # 0 and Pin # 1, as shown in the below figure:
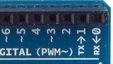
- In the Arduino Serial Read, we have seen that How to read data coming from the serial port and we have used Pin # 0 for that purpose.
- So, now we are going to write some data on the Serial Port.
- It's like we are sending data from Arduino to some other device via Serial Port.
- For example, you are using a GSM module with Arduino then you have to send AT commands to your GSM board from Arduino and that's where you use Arduino Serial write.
- You can download the Proteus Simulation and code for Arduino Serial Write Command by clicking the below button:
- Here's the first syntax for Arduino Serial write:
- Arduino Serial Write is used to write some data on the Serial Port and it sends data in binary form.
- Here's Arduino Serial Write Syntax:
Serial.write ( 'DataSent' ) ;
where:
- DataSent is a simple byte and is used in these characters ' '. The below example code will send byte '1' on the serial port:
Serial.write ( '1' ) ;
- Now, let's write some data on Arduino Serial Port using the above syntax and see what we got.
Proteus Simulation
- So, design a Proteus Simulation as shown in the below figure:
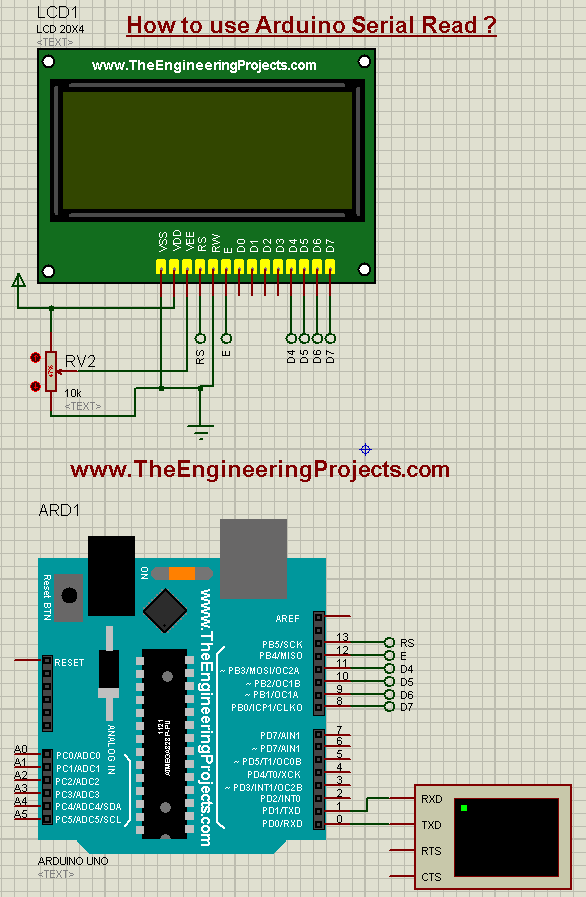
- Upload the below code in your Arduino software and get the Hex File from Arduino.
#include <LiquidCrystal.h> // initialize the library with the numbers of the interface pins LiquidCrystal lcd(13, 12, 11, 10, 9, 8); void setup() { // set up the LCD's number of columns and rows: lcd.begin(20, 4); // Print a message to the LCD. lcd.setCursor(1,0); lcd.print("www.TheEngineering"); lcd.setCursor(4,1); lcd.print("Projects.com"); lcd.setCursor(1,0); Serial.begin(9600); lcd.clear(); Serial.write('1'); } void loop() { }
- In the above code, I have simply written a byte which you can see is 1.
- So, now upload it and run your simulation and if everything goes fine then you will get 1 on your virtual serial terminal of Proteus, as shown in the below figure:
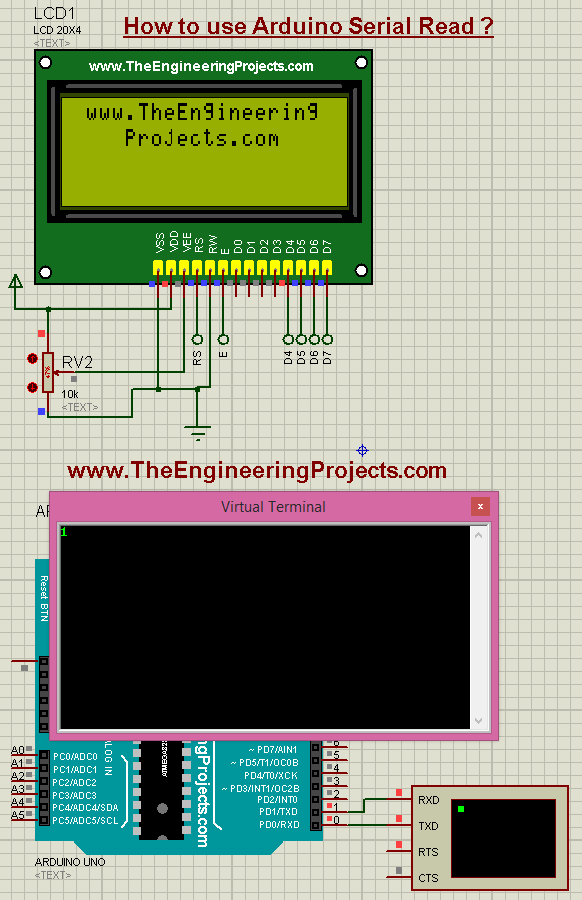
- You can see in the above figure that we got 1 in Serial Port so now you can send whatever you want via this Arduino Serial Write Command.
- Now let's have a look at the second syntax of the Arduino Serial Write command:
- We can also send a String of bytes via Arduino Serial Write Command. Here's the syntax:
Serial.write ( "DataSent" ) ;
where:
- DataSent is a simple byte and is used in these characters " ". The below example code will send our site address on the serial port:
Serial.write ( "www.TheEngineeringProjects.com" ) ;
- Now let's sent a string of bytes through this Arduino Serial Write Command, so I have used the below code and have sent our website address via Serial Write.
- So, use the below code and get your Hex File:
#include <LiquidCrystal.h> // initialize the library with the numbers of the interface pins LiquidCrystal lcd(13, 12, 11, 10, 9, 8); void setup() { // set up the LCD's number of columns and rows: lcd.begin(20, 4); // Print a message to the LCD. lcd.setCursor(1,0); lcd.print("www.TheEngineering"); lcd.setCursor(4,1); lcd.print("Projects.com"); lcd.setCursor(1,0); Serial.begin(9600); // lcd.clear(); Serial.write("www.TheEngineeringProjects.com"); } void loop() { }
- Run your Proteus Simulation and you will get the below results:
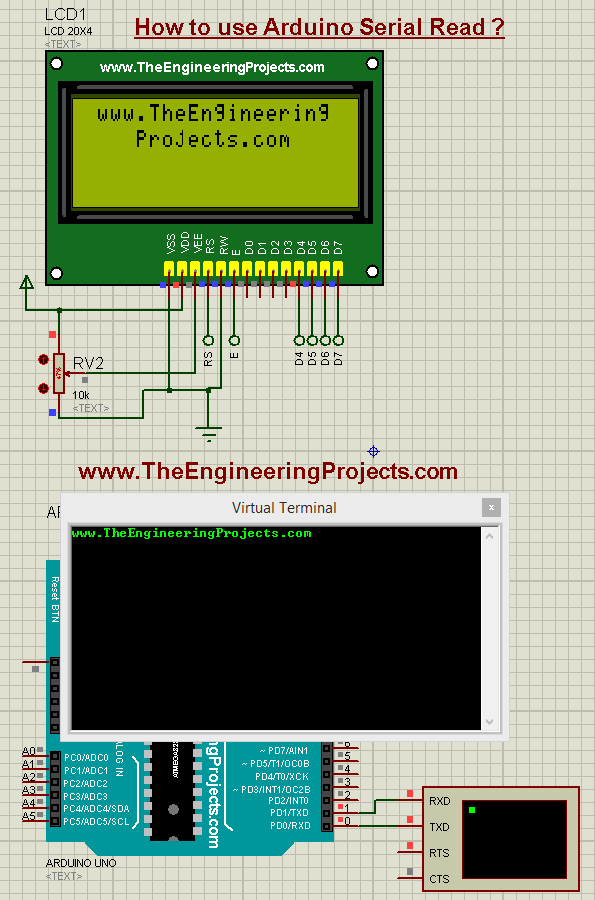
- You can see in the above figure that we got the whole address via Serial Port.